Python - for Loop
Till now, we have learned and executed sequential statements. Sometimes we want to do the same task a fixed number of times. This is done by using Looping statements.
Loop control statement is also known as repetition statements or iteration statements.
Python supports the following Loop control flow statements.
- for loop
- while loop
The for loop
A for loop usually iterates over sequential data types such as string, list, tuple, set, or dictionary.
Syntax of Python for loop
for variable_name in sequence_type:
Statement(s)
The for loop executes a set of statements once for each item in the sequence type.
Flow Chart
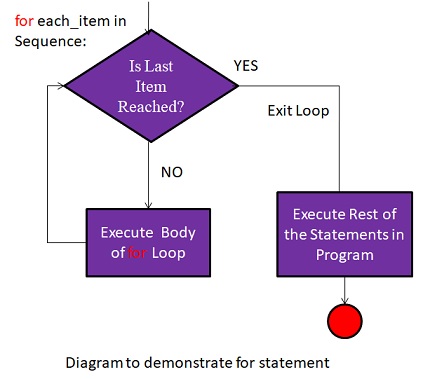
Python for Loop Example
The following example uses the for loop to display the items from the Agelist.
For example:
>> Agelist=[20,30,40,50]
>> for a in Agelist:
... print(a)
...
When you execute the above program, you will get the following output.
...
20
30
40
50
>>
Sometimes we want to display the index of each member in the list. We can do that by using enumerate() function.
>> Agelist=[20,30,40,50]
>> for In_Agelist in enumerate(Agelist):
... print(In_Agelist)
...
While executing the above code, you will get the following output.
...
(0, 20)
(1, 30)
(2, 40)
(3, 50)
>>
The range() Function
- In for loop, the range() function is used in the place of sequence to specify the initial, final, and incremental values for iteration.
- The range() function generates the lists that start from start to till stop-1.
- The syntax of the range() function is.
range([start],stop,[step])
Example with range() Function
Following is an example to demonstrate the range() function.
print("To speify the stop only argument:")
for i in range(5):
print("The value of i is: ",i)
print("To specify both start and stop arguments:")
for i in range(4,10):
print("The value of i is: ",i)
print("To specify all three arguments:")
for i in range(1,8,2):
print("The value of i is: ",i)
While executing the above code, you will get the following output.
F:\>python sample1.py
To speify the stop only argument:
The value of i is: 0
The value of i is: 1
The value of i is: 2
The value of i is: 3
The value of i is: 4
To specify both start and stop arguments:
The value of i is: 4
The value of i is: 5
The value of i is: 6
The value of i is: 7
The value of i is: 8
The value of i is: 9
to specify all three arguments:
The value of i is: 1
The value of i is: 3
The value of i is: 5
The value of i is: 7