Python - while Loop
In python, the while loop is used to execute the block or group of statements repeatedly as long as the condition is True.
Syntax of Python while statement
while conditional_expression:
Statement (S)
- The syntax starts with a while keyword and follows a condition and colon
- The condition is an expression which returns Boolean values True or False.
- It consists of a block of statements.
- The while loop first evaluates the condition that if it is True, then the loop block will be executed. After the first execution, the conditional expression is evaluated again, if it is True, then the block is executed again.
- This process is repeated until the condition is False.
- If the condition is False, the loop will be ended.
- Then, the execution continues with the first statement after the while loop.
- Each repetition of the loop block is called an iteration of the loop.
Flow Chart
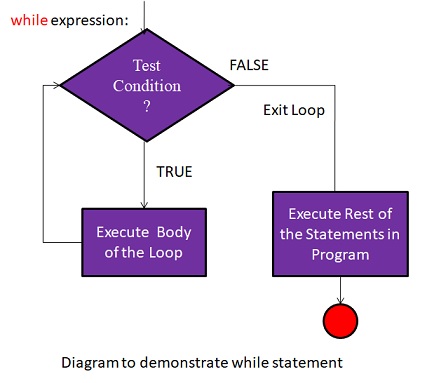
Example with Python while loop
>> sum=0
>> while sum<10:
... print("The value of sum is",sum)
... sum=sum+1
...
when you execute the above program , you will get the folloing output.
The value of sum is 0
The value of sum is 1
The value of sum is 2
The value of sum is 3
The value of sum is 4
The value of sum is 5
The value of sum is 6
The value of sum is 7
The value of sum is 8
The value of sum is 9
Python while loop with else
- In python, the while loop uses the else part within the loop.
- The else part is an optional one that contains the set of statements.
- The else part is executed if the condition is false.
Syntax of python while loop with else
while conditional_expression:
Statement (S)
else: # optional block
Statements(s)
Example - Python while loop with else
Following is an example for using else part within while loop.
i=10 # initialize the control variable
print("The value of i:")
while(i<=20): # to check condition
print(i,end="\t") # to execute the statement block
i=i+1 # to update the control variable
else:
print("\n The final value of i: ",i)
when you execute the above program, you will get the folloing output.
F:\>python sample.py
The value of i:
10 11 12 13 14 15 16 17 18 19 20
The final value of i: 21