C for loops, C and C++ for loop example
A for loop in C programming is used to execute a block of code or part of program several times.
- for loop can be embedded inside another for loop, while loop or do while loop
- for loop can start from a specific value and can modify the value to use at each iteration
- for loop can stop processing at a particular value
- for loop can invoke functions in c programming within the block
for loop syntax in C programming
for loop in C has a general form
for(expr1 ; expr2; expr3)
{
statements;
}
- The for loop has an optional expr1, expr2 and expr3 but the semicolon is compulsary.
- The expr1 is used to initialize the control variable and it is executed only once.
- The expr2 is a condition that is to be evaluated.
- If the condition is false, then the for loop is terminated.
- If the condition is true, the code inside the for loop body will be executed.
- The expr3 is a statement that is updated every execution. Usually,this expression is a increment or decrement but can be any expression. This process will repeat until the condition expr2 becomes false.
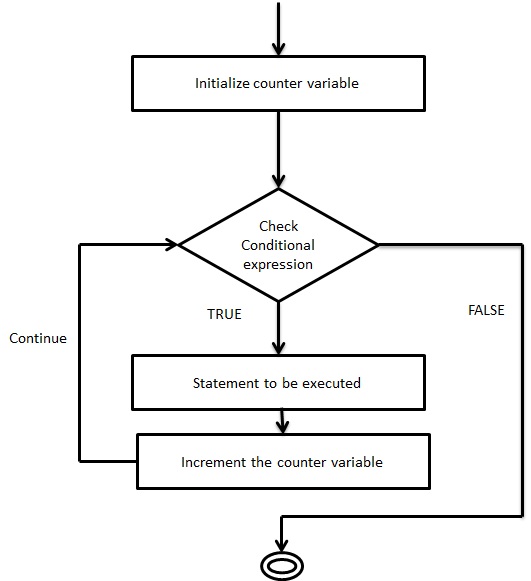
Note:
If the expr1 and expr3 are omitted, then this for loop behaves like while loop.
If the expr2 is omitted, then the condition is always TRUE and we have an infinity loop as result.
for ( ; ; ) /*infinity loop */ { statements; }
Example C Program using for loop
#include<stdio.h> int main() { int a; for ( a = 0; a < 5; a++) { printf("The value of a = %d\n",a); } printf("The final value of a = %d\n",a); return(0); }
Output
When you compile and execute the above program, you will get the following output:
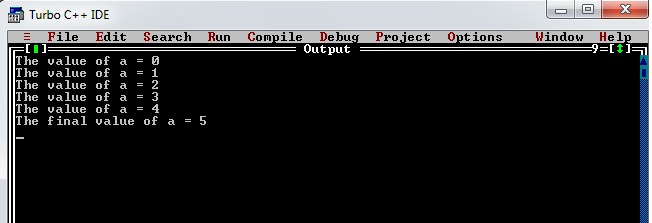