C/C++ Programming - while loop - Syntax and Example
The syntax of while loop
while(expression) statement; (or) while(expression) { statements; }
Here, first the expression is evaluated. If the conditional expression is true, statements inside the while loop will be executed after which the expression is reevaluated. This process continues until the conditional expression is false. When the condition is false, the while loop will be terminated.
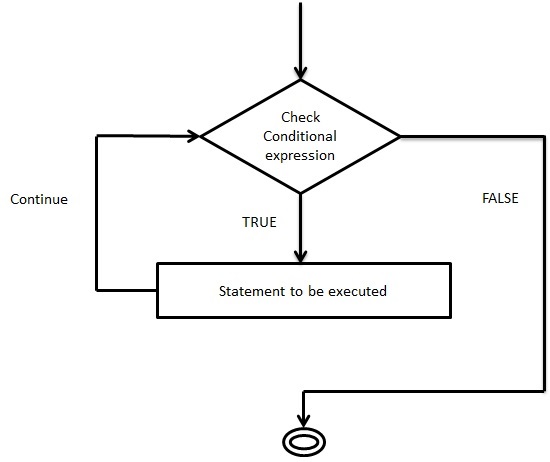
Example C Program using while loop
#include<stdio.h> int main() { int b = 0; while(b <= 5) { printf("The value of b = %d\n",b); b++ ; } printf("The final value of b = %d\n",b); return(0); }
When you compile and execute the above program, then you will get the following output:
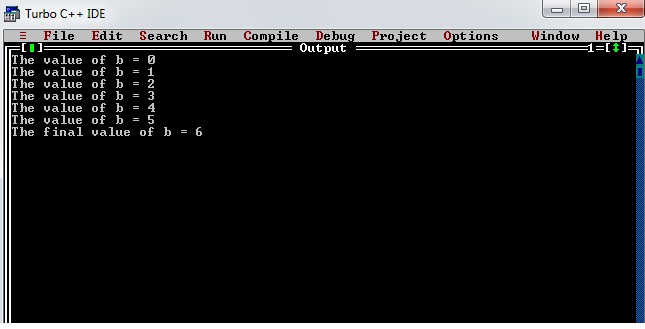