C and C++ programming If Statement
The if statement can execute the single or block of code only if the condition is true. If the condition is false, it just skips what is within the braces and continues to execute the next statement after the if block.
Syntax
if (conditional_expression){ statement1; statement2; }You can also use:
if(conditional_expression) statement;
Here, the conditional_expression is usually some kind of comparison such as relational, logical expression or bitwise expression. The condition returns a value either true or false. If the condition returns a true value, then the single statement or the block of statements will be executed. Otherwise it will be ignored. The if statement can provide the user with a very limited kind of decision. That means, it only consider the true condition. Opening and closing brace are optional only if the block contains single statement.
Flow Chart
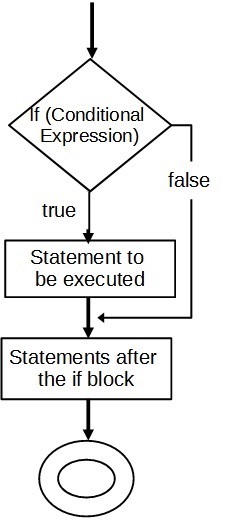
Example C Program for if statement
#include <stdio.h> #include <conio.h> int main() { int A=26; int B=30; if (A > B){ printf("The A value is greater than B \n"); A = A + 5; } if (A < B){ printf("The A value is less than B \n"); A = A - 5; } printf("The A value is: %d \n The B value is :%d",A,B); return(0) }
Output
The A value is less than B The A value is:21 The B value is:30