SC C++ Structure struct Programming, defintion, syntax and examples C
We can use array for storing multiple value in single variable-name but it can store similar data type. Sometimes, we want to have multiple data types that will be treated as whole. For example, employee record. For each record, we want to track name (character array), age(integer), address(character array), salary(floating-point), city(character array). In order to handle these type of information, C provides a special feature that is structure.
What is a structure or struct in C/C++ programming?
SThe structure is a collection of one or more variables of different data types that are grouped under a single name. By using structure, we can make a group of variables, arrays, and pointers. The structure is a user-defined data type that contains different types of variables. Each variable is known as a member of the structure.
Defining a Structure in C/C++
The struct keyword is used to define the structure. Let us see the syntax of the structure that is given below
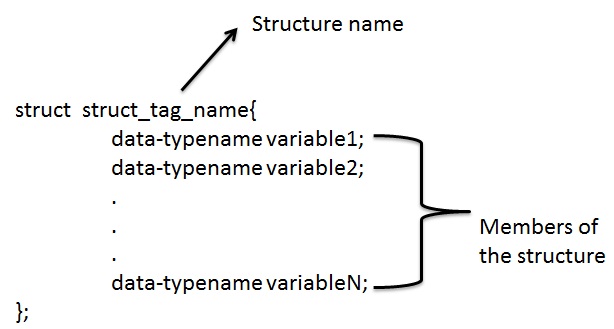
For example:
struct Employee{ char name[20]; int age; float salary; };
Here employee is the structure name and this contains three variables such as name,age and salary which are called members of the employee structure.
Note:
First letter of structure_tag_name should always be named with upper case. This distinguishes them from other variable and function and other symbolic constant.
Declaring Structure Variable
In order to access the member of the structure, we need to declare the structure variable. Following is the syntax to declare structure variable.
struct struct_tag_name structure_variable;
This is written inside the main() function.
For example:
Let us consider the above employee structure to declare structure variable.
struct Employee emp1;
We can also declare more than one structure variables that are separated by a comma. The following statement is written inside the main() function.
struct Employee emp1,emp2,emp3;
It is also possible to declare the structure variable at the time of the structure's definition. In this case, structure variables are defined at the end of the structure's definition before the semicolon.
struct Employee{ char name[20]; int age; float salary; }emp1,emp2;
Accessing Structure's Members in C/C++
To access an individual member of structure, we can use special operator that is called dot operator. The dot operator is included between the structure's variable and member of the structure. The format of the dot operator is given below.
structure_variable . member_name;
For example:
Let us put the age of the 1st employee.
emp1. Age =20;
Example program using C/C++ structure.
#include<stdio.h> /*define Student structure */ struct Student { int id; char name[20]; char branch[10]; float percentage; }; void main() { struct Student S1; //declare S1 variable for Student structure clrscr(); //initialize member of the Student structure S1.id = 1; strcpy(S1.name, "krishna"); strcpy(S1.branch, "CSE"); S1.percentage = 78.8; //print the student details printf("\n The student id is: %d ",S1.id); printf("\n The name is: %s ",S1.name); printf("\n The branch is: %s ",S1.branch); printf("\n The percentage is: %f% ",S1.percentage); }
Outpuut
The student id is: 1 The name is: krishna The branch is: CSE The percentage is: 78.80%
strcat() - String Concatenation strcmp() - String Compare
strcpy() - String Copy strlen() - String Length