C recursion function - Factorial Number Example
The functions in C are recursive which means that they can call themselves. The act of self reference is known as recursion. The function that contains itself and call itself is called recursive function. Sometimes, the recursive function is also known as tail recursion.
C Recursion function - General Form:
recursiveFunction(){ //other statements recursiveFunction(); }
C Recursion function - Example
Let us understand the recursive function from the following example program:
#include<stdio.h> int factorial(int m); void main() { int n, s; printf( "Enter input value:"); scanf("%d",&n); s = factorial(n); printf("\nThe result is: %d",s); getch(); } int factorial(int n) { int result; if(n < 0) return -1; if(n == 0) return 1; result = n * factorial(n - 1); return(result); }
Output:
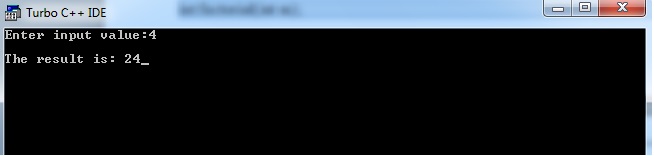
strcat() - String Concatenation strcmp() - String Compare
strcpy() - String Copy strlen() - String Length