C and C++ Goto Statement - Jump statement
The goto statement is used to simply change the normal flow of a program.
- goto statement jumps the program control to the specified label.
- The label is an identifier that occurs somewhere else in the given function and is followed by a colon.
- Labels have their namespace so that they cannot clash with the name of the variables and functions.
You can use any label like:
- here
- start
- end
- before calling
goto statement in C and C++ - Syntax
goto Label; . . . . . Label:
Using goto statement in C and C++ programming is a poor programming style. goto statement should be avoided as far as possible. C and C++ programming offers all possible ways to control execution like if statements, for loops, while loops, do while loop, switch case statements, break statement, continue statement, return statement, and try-catch blocks, function calls to control flows. Hence, goto can always be avoided in C and C++ programming. Understanding how goto statement works will help understand millions of software code written over several decades and still remain running in some enterprise. For new programs written, goto should be avoided
goto Statement - C Program Example
#include<stdio.h> int main() { int a; for(a = 0 ; a<5 ; a++) { if(a == 3) goto step; printf("The value of a = %d\n",a); } step: a = a * 2; printf("After using goto, The value of a = %d\n",a); return(0); }
goto Statement Example - Program Output
When you compile and execute the above program, then you will get the following output:
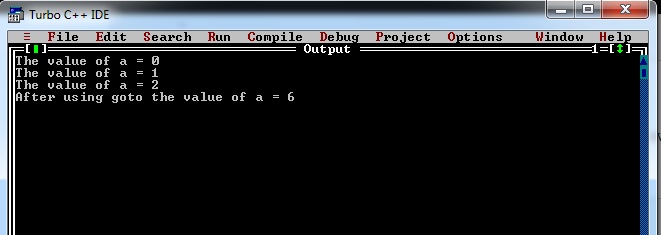