do while loop in C and C++ - Example Program
Syntax of do while
do
{
statements;
}while(expression);
do while loop has similar behavior as while loop but it has one difference. do while loop always executes the statements at least once. First the block of code is executed then the conditional expression is evaluated. If the expression is true, then the block of code is executed again. This process will continue until the conditional expression is false. when it is false, the loop will be terminated and the control of the program jumps to the next statement after the while statement.
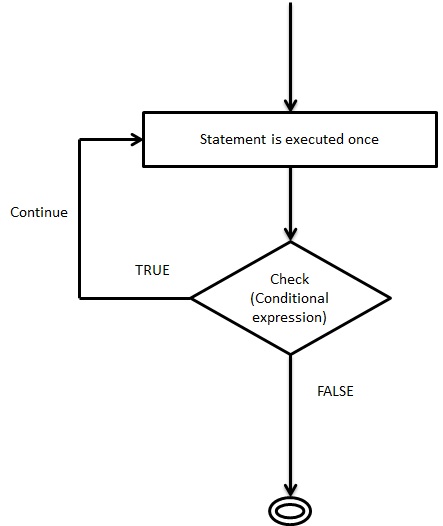
Example C Program using do...while loop
#include<stdio.h> int main() { int c = 0; do { printf("The value of c = %d\n",c); c++ ; }while(c > 5); printf("The final value of c = %d\n",c); return(0); }
Output
When you compile and execute the above program, you will get the following output:
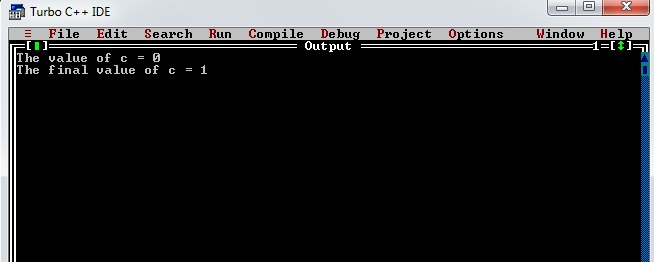